OpenAlex API in Python#
by Michael T. Moen
OpenAlex API Documentation: https://docs.openalex.org/
OpenAlex API License: https://docs.openalex.org/additional-help/faq#how-is-openalex-licensed
The OpenAlex API is licensed under the Creative Commons’ CC0 license, designating its data as part of the public domain.
These recipe examples were tested January 22, 2024.
NOTE: The OpenAlex API limits requests to a maximum of 100,000 per user per day and 10 requests per second.
Setup#
API User Information#
An API key is not required to access the OpenAlex API. However, it is recommended that you add your email to all API requests. Doing so will provide much faster and much more consistent response times.
email = 'example@domain.com'
Alternatively, you can save the above data in a separate python file and import it:
from user_info import email
OpenAlex Snapshot#
OpenAlex also offers a database download that is updated monthly. This option may be more useful than the API when working with larger datasets and when up-to-date data is unimportant.
More information can be found here.
Import Libraries#
This tutorial uses the following libraries:
import requests # Manages API requests
import matplotlib.pyplot as plt # Creates visualization of data
from time import sleep # Allows staggering of API requests to conform to rate limits
from collections import Counter # Creates dictionaries from counting elements in a list
1. Simple queries#
The OpenAlex API has a works
endpoint that can be used to search for works using a query. In this example, we’ll consider several different queries. More information on search queries can be found here: https://docs.openalex.org/how-to-use-the-api/get-lists-of-entities/search-entities
Simple title query#
This first example will search for the term “geodetics”:
endpoint = 'works'
filters = 'title.search:"geodetics"'
url = f'https://api.openalex.org/{endpoint}?filter={filters}&mailto={email}'
geodetics_data = requests.get(url).json()
# Display resulting metadata
geodetics_data['meta']
{'count': 12361,
'db_response_time_ms': 104,
'page': 1,
'per_page': 25,
'groups_count': None}
The ‘count’ in the output above tells us how many works have been published by UA authors.
It’s also important to note that the data returned by this API request is just the first page of size 25. That means that in order to obtain the data for all works in data_retrieved['results']
, we must page through this data.
Each work obtained in the query will have the following structure:
geodetics_data['results'][0]
{'id': 'https://openalex.org/W2097951601',
'doi': 'https://doi.org/10.1002/2014gc005407',
'title': 'A geodetic plate motion and Global Strain Rate Model',
'display_name': 'A geodetic plate motion and Global Strain Rate Model',
'relevance_score': 292.1121,
'publication_year': 2014,
'publication_date': '2014-10-01',
'ids': {'openalex': 'https://openalex.org/W2097951601',
'doi': 'https://doi.org/10.1002/2014gc005407',
'mag': '2097951601'},
'language': 'en',
'primary_location': {'is_oa': False,
'landing_page_url': 'https://doi.org/10.1002/2014gc005407',
'pdf_url': None,
'source': {'id': 'https://openalex.org/S14326618',
'display_name': 'Geochemistry Geophysics Geosystems',
'issn_l': '1525-2027',
'issn': ['1525-2027'],
'is_oa': True,
'is_in_doaj': True,
'host_organization': 'https://openalex.org/P4310320503',
'host_organization_name': 'Wiley-Blackwell',
'host_organization_lineage': ['https://openalex.org/P4310320503',
'https://openalex.org/P4310320595'],
'host_organization_lineage_names': ['Wiley-Blackwell', 'Wiley'],
'type': 'journal'},
'license': None,
'version': None,
'is_accepted': False,
'is_published': False},
'type': 'article',
'type_crossref': 'journal-article',
'indexed_in': ['crossref'],
'open_access': {'is_oa': False,
'oa_status': 'closed',
'oa_url': None,
'any_repository_has_fulltext': False},
'authorships': [{'author_position': 'first',
'author': {'id': 'https://openalex.org/A5063283012',
'display_name': 'C. Kreemer',
'orcid': 'https://orcid.org/0000-0001-6882-9809'},
'institutions': [{'id': 'https://openalex.org/I134113660',
'display_name': 'University of Nevada Reno',
'ror': 'https://ror.org/01keh0577',
'country_code': 'US',
'type': 'education',
'lineage': ['https://openalex.org/I134113660',
'https://openalex.org/I68260882']}],
'countries': ['US'],
'is_corresponding': False,
'raw_author_name': 'Corné Kreemer',
'raw_affiliation_string': 'Nevada Bureau of Mines and Geology, University of Nevada; Reno Nevada USA',
'raw_affiliation_strings': ['Nevada Bureau of Mines and Geology, University of Nevada; Reno Nevada USA']},
{'author_position': 'middle',
'author': {'id': 'https://openalex.org/A5024627725',
'display_name': 'G. Blewitt',
'orcid': 'https://orcid.org/0000-0002-7490-5983'},
'institutions': [{'id': 'https://openalex.org/I134113660',
'display_name': 'University of Nevada Reno',
'ror': 'https://ror.org/01keh0577',
'country_code': 'US',
'type': 'education',
'lineage': ['https://openalex.org/I134113660',
'https://openalex.org/I68260882']}],
'countries': ['US'],
'is_corresponding': False,
'raw_author_name': 'Geoffrey Blewitt',
'raw_affiliation_string': 'Nevada Bureau of Mines and Geology, University of Nevada; Reno Nevada USA',
'raw_affiliation_strings': ['Nevada Bureau of Mines and Geology, University of Nevada; Reno Nevada USA']},
{'author_position': 'last',
'author': {'id': 'https://openalex.org/A5059073242',
'display_name': 'E. Klein',
'orcid': 'https://orcid.org/0000-0003-3239-5118'},
'institutions': [{'id': 'https://openalex.org/I134113660',
'display_name': 'University of Nevada Reno',
'ror': 'https://ror.org/01keh0577',
'country_code': 'US',
'type': 'education',
'lineage': ['https://openalex.org/I134113660',
'https://openalex.org/I68260882']},
{'id': 'https://openalex.org/I4210104594',
'display_name': 'AIR Worldwide (United States)',
'ror': 'https://ror.org/00xr96s24',
'country_code': 'US',
'type': 'company',
'lineage': ['https://openalex.org/I4210104594']}],
'countries': ['US'],
'is_corresponding': False,
'raw_author_name': 'Elliot C. Klein',
'raw_affiliation_string': 'Nevada Bureau of Mines and Geology, University of Nevada; Reno Nevada USA; Now at AIR-Worldwide; Boston Massachusetts USA',
'raw_affiliation_strings': ['Nevada Bureau of Mines and Geology, University of Nevada; Reno Nevada USA',
'Now at AIR-Worldwide; Boston Massachusetts USA']}],
'countries_distinct_count': 1,
'institutions_distinct_count': 2,
'corresponding_author_ids': [],
'corresponding_institution_ids': [],
'apc_list': {'value': 0,
'currency': 'USD',
'value_usd': 0,
'provenance': 'doaj'},
'apc_paid': {'value': 0,
'currency': 'USD',
'value_usd': 0,
'provenance': 'doaj'},
'has_fulltext': True,
'fulltext_origin': 'ngrams',
'cited_by_count': 600,
'cited_by_percentile_year': {'min': 99, 'max': 100},
'biblio': {'volume': '15',
'issue': '10',
'first_page': '3849',
'last_page': '3889'},
'is_retracted': False,
'is_paratext': False,
'keywords': [{'keyword': 'geodetic plate motion', 'score': 0.7904},
{'keyword': 'strain', 'score': 0.3053}],
'concepts': [{'id': 'https://openalex.org/C127313418',
'wikidata': 'https://www.wikidata.org/wiki/Q1069',
'display_name': 'Geology',
'level': 0,
'score': 0.8476205},
{'id': 'https://openalex.org/C58754882',
'wikidata': 'https://www.wikidata.org/wiki/Q1502887',
'display_name': 'Geodetic datum',
'level': 2,
'score': 0.7491107},
{'id': 'https://openalex.org/C13280743',
'wikidata': 'https://www.wikidata.org/wiki/Q131089',
'display_name': 'Geodesy',
'level': 1,
'score': 0.72706705},
{'id': 'https://openalex.org/C149342994',
'wikidata': 'https://www.wikidata.org/wiki/Q3181055',
'display_name': 'Strain rate',
'level': 2,
'score': 0.57081014},
{'id': 'https://openalex.org/C119477230',
'wikidata': 'https://www.wikidata.org/wiki/Q7950',
'display_name': 'Plate tectonics',
'level': 3,
'score': 0.56601644},
{'id': 'https://openalex.org/C104114177',
'wikidata': 'https://www.wikidata.org/wiki/Q79782',
'display_name': 'Motion (physics)',
'level': 2,
'score': 0.5647442},
{'id': 'https://openalex.org/C2778022156',
'wikidata': 'https://www.wikidata.org/wiki/Q576145',
'display_name': 'Strain (injury)',
'level': 2,
'score': 0.48464948},
{'id': 'https://openalex.org/C165205528',
'wikidata': 'https://www.wikidata.org/wiki/Q83371',
'display_name': 'Seismology',
'level': 1,
'score': 0.43798384},
{'id': 'https://openalex.org/C154945302',
'wikidata': 'https://www.wikidata.org/wiki/Q11660',
'display_name': 'Artificial intelligence',
'level': 1,
'score': 0.08199021},
{'id': 'https://openalex.org/C77928131',
'wikidata': 'https://www.wikidata.org/wiki/Q193343',
'display_name': 'Tectonics',
'level': 2,
'score': 0.06480235},
{'id': 'https://openalex.org/C41008148',
'wikidata': 'https://www.wikidata.org/wiki/Q21198',
'display_name': 'Computer science',
'level': 0,
'score': 0.05638081},
{'id': 'https://openalex.org/C71924100',
'wikidata': 'https://www.wikidata.org/wiki/Q11190',
'display_name': 'Medicine',
'level': 0,
'score': 0.0},
{'id': 'https://openalex.org/C192562407',
'wikidata': 'https://www.wikidata.org/wiki/Q228736',
'display_name': 'Materials science',
'level': 0,
'score': 0.0},
{'id': 'https://openalex.org/C126322002',
'wikidata': 'https://www.wikidata.org/wiki/Q11180',
'display_name': 'Internal medicine',
'level': 1,
'score': 0.0},
{'id': 'https://openalex.org/C191897082',
'wikidata': 'https://www.wikidata.org/wiki/Q11467',
'display_name': 'Metallurgy',
'level': 1,
'score': 0.0}],
'mesh': [],
'locations_count': 1,
'locations': [{'is_oa': False,
'landing_page_url': 'https://doi.org/10.1002/2014gc005407',
'pdf_url': None,
'source': {'id': 'https://openalex.org/S14326618',
'display_name': 'Geochemistry Geophysics Geosystems',
'issn_l': '1525-2027',
'issn': ['1525-2027'],
'is_oa': True,
'is_in_doaj': True,
'host_organization': 'https://openalex.org/P4310320503',
'host_organization_name': 'Wiley-Blackwell',
'host_organization_lineage': ['https://openalex.org/P4310320503',
'https://openalex.org/P4310320595'],
'host_organization_lineage_names': ['Wiley-Blackwell', 'Wiley'],
'type': 'journal'},
'license': None,
'version': None,
'is_accepted': False,
'is_published': False}],
'best_oa_location': None,
'sustainable_development_goals': [{'id': 'https://metadata.un.org/sdg/13',
'display_name': 'Climate action',
'score': 0.17},
{'id': 'https://metadata.un.org/sdg/11',
'display_name': 'Sustainable cities and communities',
'score': 0.11}],
'grants': [],
'referenced_works_count': 362,
'referenced_works': ['https://openalex.org/W20396956',
'https://openalex.org/W156434746',
'https://openalex.org/W1484450406',
'https://openalex.org/W1508833321',
'https://openalex.org/W1515556863',
'https://openalex.org/W1519264343',
'https://openalex.org/W1519872695',
'https://openalex.org/W1533347802',
'https://openalex.org/W1539959491',
'https://openalex.org/W1559725960',
'https://openalex.org/W1567412536',
'https://openalex.org/W1580751720',
'https://openalex.org/W1585417129',
'https://openalex.org/W1588496391',
'https://openalex.org/W1596365679',
'https://openalex.org/W1599064168',
'https://openalex.org/W1608422347',
'https://openalex.org/W1634700694',
'https://openalex.org/W1636673817',
'https://openalex.org/W1641418782',
'https://openalex.org/W1660805619',
'https://openalex.org/W1664145982',
'https://openalex.org/W1676343945',
'https://openalex.org/W1689232968',
'https://openalex.org/W1819833965',
'https://openalex.org/W1901263200',
'https://openalex.org/W1908957110',
'https://openalex.org/W1915021902',
'https://openalex.org/W1916924843',
'https://openalex.org/W1918511379',
'https://openalex.org/W1949250275',
'https://openalex.org/W1960754841',
'https://openalex.org/W1963945134',
'https://openalex.org/W1964056785',
'https://openalex.org/W1964127207',
'https://openalex.org/W1964636616',
'https://openalex.org/W1964706587',
'https://openalex.org/W1965694483',
'https://openalex.org/W1965890893',
'https://openalex.org/W1969936638',
'https://openalex.org/W1970611664',
'https://openalex.org/W1972705099',
'https://openalex.org/W1973246145',
'https://openalex.org/W1975826710',
'https://openalex.org/W1975840213',
'https://openalex.org/W1976796651',
'https://openalex.org/W1976797497',
'https://openalex.org/W1978055661',
'https://openalex.org/W1978321269',
'https://openalex.org/W1978777291',
'https://openalex.org/W1979737794',
'https://openalex.org/W1980989662',
'https://openalex.org/W1981165981',
'https://openalex.org/W1981756035',
'https://openalex.org/W1981969378',
'https://openalex.org/W1982751998',
'https://openalex.org/W1983055326',
'https://openalex.org/W1983091118',
'https://openalex.org/W1985059677',
'https://openalex.org/W1985244050',
'https://openalex.org/W1985326935',
'https://openalex.org/W1985558613',
'https://openalex.org/W1985821678',
'https://openalex.org/W1986813420',
'https://openalex.org/W1987457357',
'https://openalex.org/W1988860700',
'https://openalex.org/W1989093648',
'https://openalex.org/W1992944908',
'https://openalex.org/W1995018844',
'https://openalex.org/W1996122598',
'https://openalex.org/W1996165282',
'https://openalex.org/W1996314941',
'https://openalex.org/W1996353541',
'https://openalex.org/W1996446456',
'https://openalex.org/W1996912040',
'https://openalex.org/W1997627183',
'https://openalex.org/W1998322169',
'https://openalex.org/W1998440704',
'https://openalex.org/W1998508127',
'https://openalex.org/W1998987137',
'https://openalex.org/W1999359239',
'https://openalex.org/W1999462066',
'https://openalex.org/W2000215114',
'https://openalex.org/W2001964436',
'https://openalex.org/W2002042240',
'https://openalex.org/W2003342124',
'https://openalex.org/W2003968851',
'https://openalex.org/W2004202092',
'https://openalex.org/W2004806678',
'https://openalex.org/W2006705681',
'https://openalex.org/W2007102529',
'https://openalex.org/W2007177347',
'https://openalex.org/W2009932337',
'https://openalex.org/W2010009527',
'https://openalex.org/W2010053969',
'https://openalex.org/W2010497141',
'https://openalex.org/W2010835919',
'https://openalex.org/W2010879384',
'https://openalex.org/W2014655678',
'https://openalex.org/W2015622589',
'https://openalex.org/W2017104286',
'https://openalex.org/W2017259013',
'https://openalex.org/W2018252114',
'https://openalex.org/W2019019057',
'https://openalex.org/W2020143845',
'https://openalex.org/W2021459991',
'https://openalex.org/W2022562942',
'https://openalex.org/W2023017648',
'https://openalex.org/W2023140651',
'https://openalex.org/W2023279549',
'https://openalex.org/W2023434032',
'https://openalex.org/W2023687553',
'https://openalex.org/W2023815011',
'https://openalex.org/W2024423630',
'https://openalex.org/W2024507466',
'https://openalex.org/W2024604632',
'https://openalex.org/W2025178195',
'https://openalex.org/W2026213263',
'https://openalex.org/W2026781522',
'https://openalex.org/W2028156169',
'https://openalex.org/W2028908386',
'https://openalex.org/W2029441462',
'https://openalex.org/W2030943620',
'https://openalex.org/W2031271302',
'https://openalex.org/W2031340793',
'https://openalex.org/W2031367151',
'https://openalex.org/W2031752895',
'https://openalex.org/W2031820423',
'https://openalex.org/W2032173908',
'https://openalex.org/W2034603228',
'https://openalex.org/W2035142411',
'https://openalex.org/W2035474168',
'https://openalex.org/W2035732572',
'https://openalex.org/W2036289410',
'https://openalex.org/W2036414886',
'https://openalex.org/W2037097477',
'https://openalex.org/W2037486556',
'https://openalex.org/W2038131649',
'https://openalex.org/W2038427352',
'https://openalex.org/W2038989587',
'https://openalex.org/W2039446227',
'https://openalex.org/W2039476948',
'https://openalex.org/W2039568461',
'https://openalex.org/W2039667389',
'https://openalex.org/W2041133524',
'https://openalex.org/W2041580614',
'https://openalex.org/W2043099620',
'https://openalex.org/W2044195310',
'https://openalex.org/W2044740267',
'https://openalex.org/W2044897744',
'https://openalex.org/W2044993690',
'https://openalex.org/W2045332374',
'https://openalex.org/W2045627460',
'https://openalex.org/W2045830288',
'https://openalex.org/W2045989154',
'https://openalex.org/W2046084095',
'https://openalex.org/W2046597214',
'https://openalex.org/W2046604344',
'https://openalex.org/W2047080222',
'https://openalex.org/W2047112069',
'https://openalex.org/W2047138213',
'https://openalex.org/W2047586898',
'https://openalex.org/W2049678959',
'https://openalex.org/W2052090465',
'https://openalex.org/W2052436825',
'https://openalex.org/W2052480187',
'https://openalex.org/W2053062298',
'https://openalex.org/W2053465043',
'https://openalex.org/W2053609724',
'https://openalex.org/W2053614592',
'https://openalex.org/W2053883225',
'https://openalex.org/W2055592383',
'https://openalex.org/W2055801959',
'https://openalex.org/W2057447701',
'https://openalex.org/W2059968538',
'https://openalex.org/W2059982963',
'https://openalex.org/W2060803307',
'https://openalex.org/W2061173674',
'https://openalex.org/W2061233675',
'https://openalex.org/W2061541952',
'https://openalex.org/W2061913696',
'https://openalex.org/W2062862625',
'https://openalex.org/W2065975709',
'https://openalex.org/W2066127711',
'https://openalex.org/W2066550063',
'https://openalex.org/W2066577158',
'https://openalex.org/W2068772241',
'https://openalex.org/W2069262562',
'https://openalex.org/W2069295925',
'https://openalex.org/W2069910165',
'https://openalex.org/W2069921444',
'https://openalex.org/W2070065272',
'https://openalex.org/W2071901145',
'https://openalex.org/W2072165976',
'https://openalex.org/W2073390790',
'https://openalex.org/W2074792146',
'https://openalex.org/W2075589505',
'https://openalex.org/W2076264905',
'https://openalex.org/W2076430169',
'https://openalex.org/W2079532029',
'https://openalex.org/W2080603404',
'https://openalex.org/W2081281319',
'https://openalex.org/W2082355668',
'https://openalex.org/W2082370467',
'https://openalex.org/W2082476880',
'https://openalex.org/W2083136661',
'https://openalex.org/W2083587239',
'https://openalex.org/W2083985973',
'https://openalex.org/W2084517204',
'https://openalex.org/W2084818772',
'https://openalex.org/W2085258952',
'https://openalex.org/W2085537191',
'https://openalex.org/W2085671464',
'https://openalex.org/W2087076405',
'https://openalex.org/W2087175177',
'https://openalex.org/W2087911315',
'https://openalex.org/W2088119000',
'https://openalex.org/W2088198088',
'https://openalex.org/W2088222709',
'https://openalex.org/W2088464272',
'https://openalex.org/W2088528510',
'https://openalex.org/W2090042005',
'https://openalex.org/W2091726787',
'https://openalex.org/W2091829898',
'https://openalex.org/W2092802330',
'https://openalex.org/W2093851945',
'https://openalex.org/W2094319079',
'https://openalex.org/W2095028869',
'https://openalex.org/W2096372699',
'https://openalex.org/W2098839042',
'https://openalex.org/W2098925389',
'https://openalex.org/W2099346524',
'https://openalex.org/W2100650518',
'https://openalex.org/W2100678637',
'https://openalex.org/W2100813995',
'https://openalex.org/W2102585420',
'https://openalex.org/W2102877934',
'https://openalex.org/W2104380822',
'https://openalex.org/W2105560525',
'https://openalex.org/W2106206390',
'https://openalex.org/W2106493024',
'https://openalex.org/W2106578909',
'https://openalex.org/W2106621358',
'https://openalex.org/W2107858435',
'https://openalex.org/W2107862835',
'https://openalex.org/W2107952396',
'https://openalex.org/W2108214772',
'https://openalex.org/W2108765362',
'https://openalex.org/W2108825923',
'https://openalex.org/W2108873720',
'https://openalex.org/W2110056895',
'https://openalex.org/W2110164758',
'https://openalex.org/W2111526563',
'https://openalex.org/W2112285521',
'https://openalex.org/W2112351610',
'https://openalex.org/W2112568224',
'https://openalex.org/W2112583448',
'https://openalex.org/W2113041309',
'https://openalex.org/W2113476263',
'https://openalex.org/W2113590693',
'https://openalex.org/W2114133266',
'https://openalex.org/W2114521104',
'https://openalex.org/W2115962899',
'https://openalex.org/W2116110625',
'https://openalex.org/W2117539158',
'https://openalex.org/W2117580125',
'https://openalex.org/W2117630863',
'https://openalex.org/W2118786662',
'https://openalex.org/W2118941360',
'https://openalex.org/W2119663013',
'https://openalex.org/W2119856988',
'https://openalex.org/W2120195262',
'https://openalex.org/W2120316772',
'https://openalex.org/W2121293282',
'https://openalex.org/W2123478882',
'https://openalex.org/W2124713962',
'https://openalex.org/W2125238892',
'https://openalex.org/W2125538700',
'https://openalex.org/W2125871441',
'https://openalex.org/W2126370198',
'https://openalex.org/W2126787697',
'https://openalex.org/W2127371491',
'https://openalex.org/W2127580561',
'https://openalex.org/W2127752167',
'https://openalex.org/W2127855430',
'https://openalex.org/W2128549739',
'https://openalex.org/W2128704751',
'https://openalex.org/W2129167994',
'https://openalex.org/W2129367996',
'https://openalex.org/W2129729729',
'https://openalex.org/W2130833734',
'https://openalex.org/W2133274607',
'https://openalex.org/W2133987485',
'https://openalex.org/W2134120211',
'https://openalex.org/W2134670327',
'https://openalex.org/W2134691634',
'https://openalex.org/W2135494568',
'https://openalex.org/W2135510402',
'https://openalex.org/W2135607818',
'https://openalex.org/W2135710208',
'https://openalex.org/W2135856981',
'https://openalex.org/W2136361999',
'https://openalex.org/W2136975928',
'https://openalex.org/W2137209222',
'https://openalex.org/W2137995611',
'https://openalex.org/W2140040222',
'https://openalex.org/W2141955097',
'https://openalex.org/W2142199997',
'https://openalex.org/W2142525864',
'https://openalex.org/W2143311695',
'https://openalex.org/W2143563294',
'https://openalex.org/W2145972115',
'https://openalex.org/W2146799928',
'https://openalex.org/W2148175431',
'https://openalex.org/W2149035079',
'https://openalex.org/W2149361682',
'https://openalex.org/W2149416836',
'https://openalex.org/W2150215708',
'https://openalex.org/W2150910670',
'https://openalex.org/W2153094327',
'https://openalex.org/W2153326760',
'https://openalex.org/W2153599025',
'https://openalex.org/W2153853022',
'https://openalex.org/W2154105358',
'https://openalex.org/W2154391437',
'https://openalex.org/W2156701839',
'https://openalex.org/W2157183908',
'https://openalex.org/W2157275807',
'https://openalex.org/W2157511539',
'https://openalex.org/W2158292240',
'https://openalex.org/W2159187621',
'https://openalex.org/W2160157470',
'https://openalex.org/W2160954014',
'https://openalex.org/W2162077393',
'https://openalex.org/W2163891561',
'https://openalex.org/W2164032877',
'https://openalex.org/W2164587882',
'https://openalex.org/W2165421589',
'https://openalex.org/W2165882798',
'https://openalex.org/W2166113849',
'https://openalex.org/W2167369680',
'https://openalex.org/W2167547540',
'https://openalex.org/W2167929451',
'https://openalex.org/W2168391315',
'https://openalex.org/W2168609878',
'https://openalex.org/W2257195885',
'https://openalex.org/W2272191557',
'https://openalex.org/W2310025584',
'https://openalex.org/W2313564179',
'https://openalex.org/W2314294150',
'https://openalex.org/W2316649687',
'https://openalex.org/W2321336637',
'https://openalex.org/W2322611715',
'https://openalex.org/W2324413234',
'https://openalex.org/W2325510633',
'https://openalex.org/W2329362906',
'https://openalex.org/W2332598638',
'https://openalex.org/W2340372422',
'https://openalex.org/W2509970959',
'https://openalex.org/W2784892447',
'https://openalex.org/W3109355539',
'https://openalex.org/W4312827208'],
'related_works': ['https://openalex.org/W4313437384',
'https://openalex.org/W130236280',
'https://openalex.org/W2375536475',
'https://openalex.org/W2412470376',
'https://openalex.org/W2062843243',
'https://openalex.org/W2369439249',
'https://openalex.org/W2349272851',
'https://openalex.org/W2964093098',
'https://openalex.org/W2963447316',
'https://openalex.org/W3020980379'],
'ngrams_url': 'https://api.openalex.org/works/W2097951601/ngrams',
'abstract_inverted_index': {'We': [0, 61, 83, 256],
'present': [1, 177, 258, 283],
'a': [2, 29, 284],
'new': [3, 178],
'global': [4, 78, 277, 285],
'model': [5, 49],
'of': [6, 38, 40, 53, 68, 128, 138, 159, 287],
'plate': [7, 13, 134, 195],
'motions': [8],
'and': [9, 230, 251, 267],
'strain': [10, 218, 222, 247],
'rates': [11],
'in': [12, 36, 44, 47, 55, 100, 145, 155, 203, 216, 262],
'boundary': [14, 214],
'zones': [15],
'constrained': [16],
'by': [17, 51, 74, 120, 152],
'horizontal': [18],
'geodetic': [19],
'velocities.': [20, 181],
'This': [21],
'Global': [22],
'Strain': [23],
'Rate': [24],
'Model': [25],
'(GSRM': [26],
'v.2.1)': [27],
'is': [28, 106, 141, 163, 206, 225],
'vast': [30],
'improvement': [31],
'over': [32],
'its': [33],
'predecessor': [34],
'both': [35],
'terms': [37],
'amount': [39],
'data': [41, 59, 125],
'input': [42],
'as': [43, 165, 213],
'an': [45, 238],
'increase': [46],
'spatial': [48],
'resolution': [50],
'factor': [52],
'∼2.5': [54],
'areas': [56, 263],
'with': [57, 190, 264, 275],
'dense': [58],
'coverage.': [60],
'determined': [62],
'6739': [63],
'velocities': [64, 86, 99, 111, 129, 253],
'from': [65, 87, 112, 245],
'time': [66, 115],
'series': [67],
'(mostly)': [69, 89],
'continuous': [70],
'GPS': [71],
'measurements;': [72],
'i.e.,': [73, 123],
'far': [75],
'the': [76, 101, 132, 139, 160, 184, 191, 201, 217, 228, 269],
'largest': [77],
'velocity': [79, 205, 241, 278],
'solution': [80, 95],
'to': [81, 96, 108, 143, 236],
'date.': [82],
'transformed': [84],
'15,772': [85],
'233': [88],
'published': [90],
'studies': [91],
'onto': [92],
'our': [93, 276],
'core': [94],
'obtain': [97, 237],
'22,511': [98],
'same': [102],
'reference': [103, 272],
'frame.': [104],
'Care': [105],
'taken': [107],
'not': [109],
'use': [110],
'stations': [113],
'(or': [114],
'periods)': [116],
'that': [117, 186, 200],
'are': [118, 211, 254],
'affected': [119],
'transient': [121],
'phenomena;': [122],
'this': [124],
'set': [126],
'consists': [127],
'best': [130],
'representing': [131, 169],
'interseismic': [133],
'velocity.': [135],
'About': [136],
'14%': [137],
'Earth': [140],
'allowed': [142],
'deform': [144],
'145,086': [146],
'deforming': [147],
'grid': [148],
'cells': [149],
'(0.25°': [150],
'longitude': [151],
'0.2°': [153],
'latitude': [154],
'dimension).': [156],
'The': [157, 208, 221],
'remainder': [158],
"Earth's": [161],
'surface': [162],
'modeled': [164, 226],
'rigid': [166],
'spherical': [167],
'caps': [168],
'50': [170],
'tectonic': [171],
'plates.': [172],
'For': [173, 182],
'36': [174],
'plates': [175, 185],
'we': [176, 198, 282],
'GPS-derived': [179],
'angular': [180, 204],
'all': [183],
'can': [187],
'be': [188],
'compared': [189],
'most': [192],
'recent': [193],
'geologic': [194],
'motion': [196],
'model,': [197],
'find': [199],
'difference': [202],
'significant.': [207],
'rigid-body': [209],
'rotations': [210],
'used': [212],
'conditions': [215],
'rate': [219, 223],
'calculations.': [220],
'field': [224],
'using': [227],
'Haines': [229],
'Holt': [231],
'method,': [232],
'which': [233, 246],
'uses': [234],
'splines': [235],
'self-consistent': [239],
'interpolated': [240],
'gradient': [242, 279],
'tensor': [243],
'field,': [244],
'rates,': [248, 250],
'vorticity': [249],
'expected': [252, 259],
'derived.': [255],
'also': [257],
'faulting': [260],
'orientations': [261],
'significant': [265],
'vorticity,': [266],
'update': [268],
'no-net': [270],
'rotation': [271],
'frame': [273],
'associated': [274],
'field.': [280],
'Finally,': [281],
'map': [286],
'recurrence': [288],
'times': [289],
'for': [290],
'Mw=7.5': [291],
'characteristic': [292],
'earthquakes.': [293]},
'cited_by_api_url': 'https://api.openalex.org/works?filter=cites:W2097951601',
'counts_by_year': [{'year': 2023, 'cited_by_count': 91},
{'year': 2022, 'cited_by_count': 98},
{'year': 2021, 'cited_by_count': 101},
{'year': 2020, 'cited_by_count': 92},
{'year': 2019, 'cited_by_count': 80},
{'year': 2018, 'cited_by_count': 56},
{'year': 2017, 'cited_by_count': 32},
{'year': 2016, 'cited_by_count': 30},
{'year': 2015, 'cited_by_count': 16},
{'year': 2014, 'cited_by_count': 1}],
'updated_date': '2024-01-16T05:41:56.805705',
'created_date': '2016-06-24'}
Index out query results#
Now that we’ve obtained some data from the API, we can index out some of data from this query:
# Display the DOI of the first work returned
geodetics_data['results'][0]['doi']
'https://doi.org/10.1002/2014gc005407'
# Display the title of the first work returned
geodetics_data['results'][0]['title']
'A geodetic plate motion and Global Strain Rate Model'
# Display the primary institution of the first author of the first work returned
geodetics_data['results'][0]['authorships'][0]['institutions'][0]['display_name']
'University of Nevada Reno'
# Display titles of first 10 results
for work in geodetics_data['results'][:10]:
print(work['title'])
A geodetic plate motion and Global Strain Rate Model
Geodetic determination of relative plate motion in central California
A testing procedure for use in geodetic networks
Effect of annual signals on geodetic velocity
Short Note: A global model of pressure and temperature for geodetic applications
Inertial Navigation Systems with Geodetic Applications
Geodetic Reference System 1980
Density assumptions for converting geodetic glacier volume change to mass change
Geodetic reference system 1980
Space-geodetic estimation of the nazca-south america euler vector
Query with boolean logic#
The API also allows us to use boolean operators AND, OR, and NOT (must be capitalized) when querying for works. This example searches for works whose abstracts contain “simulation” and “chemistry” and not “medicine” or “energy”:
endpoint = 'works'
filters = 'abstract.search:("simulation" AND "chemistry") NOT ("medicine" OR "energy")'
url = f'https://api.openalex.org/{endpoint}?filter={filters}&mailto={email}'
data_retrieved = requests.get(url).json()
# Display resulting metadata
data_retrieved['meta']
{'count': 29339,
'db_response_time_ms': 124,
'page': 1,
'per_page': 25,
'groups_count': None}
2. Retrieving publications from an institution#
The OpenAlex API provides several ways to find the works published through an institution. In this tutorial, we’ll look at how to achieve this by searching with an ROR ID and an OpenAlex ID.
Searching through an ROR identifier#
The OpenAlex API allows us to access the publications from an institution through its ROR ID. To find the ROR ID for an institution, we can search for the institution here.
To see how to programmatically obtain ROR IDs for institutions, please see our Research Organization Registry cookbook tutorials.
In this example, we’ll look at the University of Alabama, which has the ROR identifier of https://ror.org/03xrrjk67
ua_ror = 'https://ror.org/03xrrjk67'
endpoint = 'works'
filters = f'institutions.ror:{ua_ror}'
url = f'https://api.openalex.org/{endpoint}?filter={filters}&mailto={email}'
ua_data = requests.get(url).json()
# Display length of data and other info
ua_data['meta']
{'count': 56435,
'db_response_time_ms': 196,
'page': 1,
'per_page': 25,
'groups_count': None}
Searching through an OpenAlex ID#
To find the OpenAlex ID of an institution, we’ll first perform an institution search:
endpoint = 'institutions'
search = 'University of Alabama'
url = f'https://api.openalex.org/{endpoint}?search={search}&mailto={email}'
institutions_search_results = requests.get(url).json()
# Display resulting metadata
institutions_search_results['meta']
{'count': 11,
'db_response_time_ms': 22,
'page': 1,
'per_page': 25,
'groups_count': None}
institutions_search_results['results'][0]
{'id': 'https://openalex.org/I32389192',
'ror': 'https://ror.org/008s83205',
'display_name': 'University of Alabama at Birmingham',
'relevance_score': 95757.98,
'country_code': 'US',
'type': 'education',
'lineage': ['https://openalex.org/I2800507078',
'https://openalex.org/I32389192'],
'homepage_url': 'http://www.uab.edu/',
'image_url': 'https://commons.wikimedia.org/w/index.php?title=Special:Redirect/file/UABirmingham%20logo.png',
'image_thumbnail_url': 'https://commons.wikimedia.org/w/index.php?title=Special:Redirect/file/UABirmingham%20logo.png&width=300',
'display_name_acronyms': ['UAB'],
'display_name_alternatives': ["Université d'alabama à birmingham"],
'repositories': [],
'works_count': 118105,
'cited_by_count': 3980166,
'summary_stats': {'2yr_mean_citedness': 3.976269055476976,
'h_index': 576,
'i10_index': 54150},
'ids': {'openalex': 'https://openalex.org/I32389192',
'ror': 'https://ror.org/008s83205',
'mag': '32389192',
'grid': 'grid.265892.2',
'wikipedia': 'https://en.wikipedia.org/wiki/University%20of%20Alabama%20at%20Birmingham',
'wikidata': 'https://www.wikidata.org/wiki/Q1472663'},
'geo': {'city': 'Birmingham',
'geonames_city_id': '4049979',
'region': None,
'country_code': 'US',
'country': 'United States',
'latitude': 33.52066,
'longitude': -86.80249},
'international': {'display_name': {'ar': 'جامعة ألاباما في برمنغهام',
'arz': 'جامعة الاباما فى برمنجهام',
'ast': "Universidá d'Alabama en Birmingham",
'azb': 'برمینقم\u200cده\u200cکی آلاباما بیلیم\u200cیوردو',
'be': 'Алабамскі ўніверсітэт у Бірмінгеме',
'ca': "Universitat d'Alabama a Birmingham",
'cs': 'University of Alabama at Birmingham',
'de': 'University of Alabama at Birmingham',
'en': 'University of Alabama at Birmingham',
'eo': 'Universitato de Alabamo ĉe Birmingham',
'es': 'Universidad de Alabama en Birmingham',
'fa': 'دانشگاه آلاباما در برمینگهم',
'fr': "université d'Alabama à Birmingham",
'ga': 'Ollscoil Alabama, Birmingham',
'he': 'אוניברסיטת אלבמה בבירמינגהם',
'it': "Università dell'Alabama a Birmingham",
'ja': 'アラバマ大学バーミンガム校',
'pl': 'University of Alabama at Birmingham',
'ru': 'Университет Алабамы в Бирмингеме',
'sv': 'University of Alabama at Birmingham',
'uk': 'Університет Алабами в Бірмінгемі',
'ur': 'یونیورسٹی آف الاباما ایٹ برمنگھم',
'zh': '阿拉巴馬大學伯明翰分校',
'zh-cn': '亚拉巴马大學伯明翰校區',
'zh-hant': '亞拉巴馬大學伯明翰校區'}},
'associated_institutions': [{'id': 'https://openalex.org/I2800507078',
'ror': 'https://ror.org/051fvmk98',
'display_name': 'University of Alabama System',
'country_code': 'US',
'type': 'education',
'relationship': 'parent'},
{'id': 'https://openalex.org/I320755355',
'ror': 'https://ror.org/053bp9m60',
'display_name': "Children's of Alabama",
'country_code': 'US',
'type': 'healthcare',
'relationship': 'related'},
{'id': 'https://openalex.org/I4210157920',
'ror': 'https://ror.org/04vzsq290',
'display_name': 'Mississippi Alabama Sea Grant Consortium',
'country_code': 'US',
'type': 'other',
'relationship': 'related'},
{'id': 'https://openalex.org/I1335695989',
'ror': 'https://ror.org/01rm42p40',
'display_name': 'University of Alabama at Birmingham Hospital',
'country_code': 'US',
'type': 'healthcare',
'relationship': 'related'}],
'counts_by_year': [{'year': 2024,
'works_count': 363,
'cited_by_count': 22737},
{'year': 2023, 'works_count': 6255, 'cited_by_count': 306782},
{'year': 2022, 'works_count': 6306, 'cited_by_count': 308845},
{'year': 2021, 'works_count': 6459, 'cited_by_count': 303914},
{'year': 2020, 'works_count': 6677, 'cited_by_count': 271538},
{'year': 2019, 'works_count': 5887, 'cited_by_count': 230976},
{'year': 2018, 'works_count': 5597, 'cited_by_count': 210492},
{'year': 2017, 'works_count': 5250, 'cited_by_count': 198096},
{'year': 2016, 'works_count': 4935, 'cited_by_count': 189898},
{'year': 2015, 'works_count': 4569, 'cited_by_count': 185546},
{'year': 2014, 'works_count': 4570, 'cited_by_count': 175061},
{'year': 2013, 'works_count': 4277, 'cited_by_count': 162477},
{'year': 2012, 'works_count': 3609, 'cited_by_count': 150844}],
'roles': [{'role': 'funder',
'id': 'https://openalex.org/F4320310431',
'works_count': 674},
{'role': 'institution',
'id': 'https://openalex.org/I32389192',
'works_count': 118105}],
'x_concepts': [{'id': 'https://openalex.org/C71924100',
'wikidata': 'https://www.wikidata.org/wiki/Q11190',
'display_name': 'Medicine',
'level': 0,
'score': 87.1},
{'id': 'https://openalex.org/C86803240',
'wikidata': 'https://www.wikidata.org/wiki/Q420',
'display_name': 'Biology',
'level': 0,
'score': 78.9},
{'id': 'https://openalex.org/C126322002',
'wikidata': 'https://www.wikidata.org/wiki/Q11180',
'display_name': 'Internal medicine',
'level': 1,
'score': 65.8},
{'id': 'https://openalex.org/C54355233',
'wikidata': 'https://www.wikidata.org/wiki/Q7162',
'display_name': 'Genetics',
'level': 1,
'score': 49.8},
{'id': 'https://openalex.org/C185592680',
'wikidata': 'https://www.wikidata.org/wiki/Q2329',
'display_name': 'Chemistry',
'level': 0,
'score': 47.3},
{'id': 'https://openalex.org/C142724271',
'wikidata': 'https://www.wikidata.org/wiki/Q7208',
'display_name': 'Pathology',
'level': 1,
'score': 42.8},
{'id': 'https://openalex.org/C55493867',
'wikidata': 'https://www.wikidata.org/wiki/Q7094',
'display_name': 'Biochemistry',
'level': 1,
'score': 40.8},
{'id': 'https://openalex.org/C15744967',
'wikidata': 'https://www.wikidata.org/wiki/Q9418',
'display_name': 'Psychology',
'level': 0,
'score': 37.3},
{'id': 'https://openalex.org/C141071460',
'wikidata': 'https://www.wikidata.org/wiki/Q40821',
'display_name': 'Surgery',
'level': 1,
'score': 30.7},
{'id': 'https://openalex.org/C121332964',
'wikidata': 'https://www.wikidata.org/wiki/Q413',
'display_name': 'Physics',
'level': 0,
'score': 28.5},
{'id': 'https://openalex.org/C134018914',
'wikidata': 'https://www.wikidata.org/wiki/Q162606',
'display_name': 'Endocrinology',
'level': 1,
'score': 26.0},
{'id': 'https://openalex.org/C118552586',
'wikidata': 'https://www.wikidata.org/wiki/Q7867',
'display_name': 'Psychiatry',
'level': 1,
'score': 24.4},
{'id': 'https://openalex.org/C203014093',
'wikidata': 'https://www.wikidata.org/wiki/Q101929',
'display_name': 'Immunology',
'level': 1,
'score': 24.3},
{'id': 'https://openalex.org/C33923547',
'wikidata': 'https://www.wikidata.org/wiki/Q395',
'display_name': 'Mathematics',
'level': 0,
'score': 24.3},
{'id': 'https://openalex.org/C41008148',
'wikidata': 'https://www.wikidata.org/wiki/Q21198',
'display_name': 'Computer science',
'level': 0,
'score': 21.3}],
'works_api_url': 'https://api.openalex.org/works?filter=institutions.id:I32389192',
'updated_date': '2024-01-22T09:13:50.599529',
'created_date': '2016-06-24'}
This query returned several universities related to the “University of Alabama”:
for institution in institutions_search_results['results']:
print(f"{institution['display_name']}: {institution['id']}")
University of Alabama at Birmingham: https://openalex.org/I32389192
University of Alabama: https://openalex.org/I17301866
University of Alabama in Huntsville: https://openalex.org/I82495205
University of South Alabama: https://openalex.org/I83809506
University of Alabama at Birmingham Hospital: https://openalex.org/I1335695989
University of Alabama System: https://openalex.org/I2800507078
University of North Alabama: https://openalex.org/I12970578
University of South Alabama Medical Center: https://openalex.org/I4210151789
University of West Alabama: https://openalex.org/I68631920
Amridge University: https://openalex.org/I2800517358
UAB Medicine: https://openalex.org/I4390039250
As we can see above, the OpenAlex ID for the University of Alabama is “https://openalex.org/I17301866” or just “I17301866”
ua_openalex_id = 'I17301866'
endpoint = 'works'
filters = f'institutions.id:{ua_openalex_id}'
url = f'https://api.openalex.org/{endpoint}?filter={filters}&mailto={email}'
ua_data = requests.get(url).json()
# Display resulting metadata
ua_data['meta']
{'count': 56435,
'db_response_time_ms': 127,
'page': 1,
'per_page': 25,
'groups_count': None}
As we can see from the count
in the metadata, these two approaches found the same number of publications.
3. Paging through the results of a query#
In the examples above, we have only retrieved the first page of results for a query. If we wish to obtain all of the data for an institution, we must page through the results. Paging is used in the example below to obtain all of the publications by UA in the OpenAlex database:
# Parameters for obtaining UA publications
endpoint = 'works'
filters = 'institutions.id:I17301866'
# In cursor paging, the cursor to the next page is given in the metadata of the response
# To get the first page, we set the cursor parameter to '*'
cursor = '*'
page_size = 200
ua_articles = []
# When the end of the results has been reached, the 'next_cursor' variable will be null
# This while loop iterates through all pages of the results
while cursor is not None:
# Set up HTTP request and request data
url = f'https://api.openalex.org/{endpoint}?filter={filters}&cursor={cursor}&per-page={page_size}&mailto={email}'
page_data = requests.get(url).json()
# Set cursor to the next page
cursor = page_data['meta']['next_cursor']
# Add results to the ua_articles list
ua_articles.extend(page_data['results'])
# Wait 0.2 seconds between requests to follow OpenAlex's rate limit
sleep(0.2)
# Display number of UA articles in database
len(ua_articles)
56435
Plotting UA publications by year#
This example plots the number of UA publications per year from 1970 onward:
# Extract publication years
publication_years = []
for article in ua_articles:
if article['publication_year'] >= 1970:
publication_years.append(article['publication_year'])
# Count occurrences of each publication year
year_counts = Counter(publication_years)
# Plot data
years = list(year_counts.keys())
counts = list(year_counts.values())
plt.bar(years, counts, color='skyblue')
plt.xlabel('Publication Year')
plt.ylabel('Number of Articles')
plt.title('UA Articles per Publication Year')
plt.show()
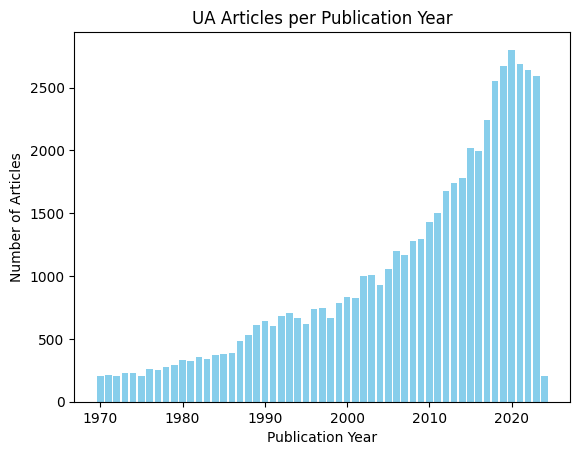
Plotting open access UA publications#
Inspired by: ourresearch/openalex-api-tutorials
This example plots the prevalence open access in UA articles from 2000 onward:
# Extract publication years and 'is_open' values
year_is_open_data = [(article['publication_year'], article['open_access']['is_oa']) for article in ua_articles]
# Filter to include only years after 1999
filtered_data = [(year, is_open) for year, is_open in year_is_open_data if 2008 <= year <= 2023]
# Count occurrences of (year, is_open)
count_per_year_is_open = Counter(filtered_data)
# Plot data
years = sorted(set(year for year, _ in filtered_data))
open_access_counts = [count_per_year_is_open[(year, True)] for year in years]
not_open_access_counts = [count_per_year_is_open[(year, False)] for year in years]
# Compute and add percentages
percent_oa = []
for oa,not_oa in zip(open_access_counts,not_open_access_counts):
percent_oa.append(round((oa/(oa + not_oa)*100)))
# Plot with percentage labels
fig, ax = plt.subplots(figsize=(10, 5))
bars = plt.bar(years, open_access_counts, color='darkgreen', label='Open Access')
# Add labels above bars
for bar, percent in zip(bars, percent_oa):
height = bar.get_height()
ax.text(bar.get_x() + bar.get_width() / 2, height, f'{percent}%', ha='center', va='bottom', color='white', fontsize=10)
plt.bar(years, not_open_access_counts, bottom=open_access_counts, color='darkred', label='Not Open Access')
plt.xlabel('Publication Year')
plt.ylabel('Number of Articles')
plt.title('Open Accss UA Articles per Publication Year')
plt.legend(loc='upper left')
plt.show()
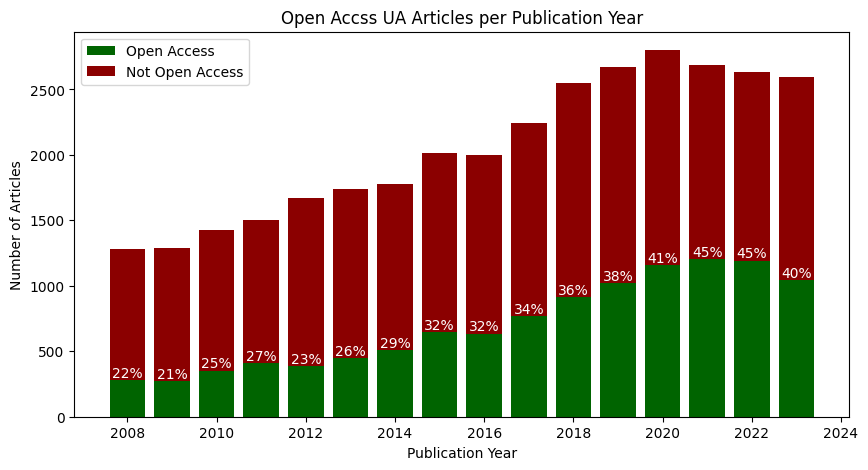